
In the previous tutorial about how to run java program code, we have successfully written, compiled and run java program code. Now, I'm going to play around with the code to see what happens if the code doesn't compile successfully.
Display Text Messages with Java
Previously, we have successfully displayed the text "Hello World". Now I want to change the contents of the file to "Pssstt ... I want to learn Java":
Here is the program code:
class HelloLearn {
public static void main(String args[]){
System.out.println("Pssstt ... I want to learn Java");
}
}
Save as HelloLearn.java. Notice, aside from the text in line 3, I also change the class name in line 1 to HelloLearn.
Just like before, we have to compile this program code using javac.exe:
javac HelloLearn.java
There is a slight change here. Besides the file name, I only write "javac", no longer "javac.exe". This is because in Windows cmd, the .exe application can be called without the word ".exe". Next, do the same thing as before for the process of running Java byte code:
java HelloLearn
As a result, the text "Pssstt ... I want to learn Java".
Just a reminder, the javac and java commands here are used to call the javac.exe and java.exe files located in the C:\Program Files\Java\JDK_VERSION\bin\ folder. However, because we have previously add java Jdk to the Windows PATH, then now, both can be called from any folder.
Check Error Messages in Java
If the program code has an error, an error message will appear during the compile process. Let's try it.
Create a new file ShowError.java with the following code:
class ShowError {
public static void main(String args[]){
System.out.println("There was a little error...")
}
}
Now, what has changed is the name of the class in line 1 and the contents of the text in line 3. Notice I accidentally deleted the semicolon at the end of line 3. The following results when compiling with javac:
Display when an error occurs. The point is, focus on the error message that appears, i.e. error: ";" expected. Here, javac.exe complains because in line 3 there should be a semicolon ";" The caret or cap "^" also indicates the position of the error detected.
If the compilation process generates an error, no java .class file will be created until the problem is resolved.
Okay, please add a semicolon at the end of line 3, then delete the quotation marks instead of the end of the text:
class ShowError {
public static void main(String args[]){
System.out.println("There was a little error...);
}
}
Save the ShowError.java file and recompile it:
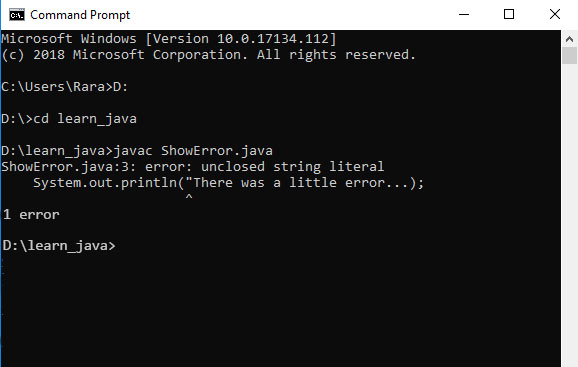
This time, the error message is an unclosed string literal. Here, javac.exe complains because there is no closed string. The cap character "^" refers to the first quotation marks, quotation marks that are not found in closing pairs. Now, try fixing it again.
Those are some of the error messages that will often be encountered during coding in Java. Java error code is a very understandable thing. Even the most expert programmers often encounter errors. As long as you can understand the error in question, it doesn't matter.
If you feel deadlocked, please copy and paste the error message to Google. Most likely there are other programmers who face similar problems and explain the solution.
Further explanation about the rules of writing Java code, I will discuss in a separate tutorial. For now, we just focus on learn to understand java error message only.
Error messages are everyday friends for the programmer, especially if we have handled complex program code and consists of tens or hundreds of lines of program code. Therefore, learn to understand the error code and find the cause.