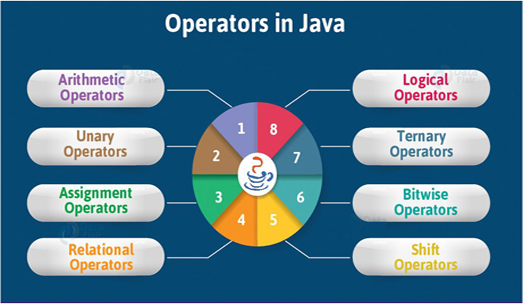
Operators in java programming are used to perform certain operations. Suppose we want to add the values of the variables x and y, then we can use the addition operator (+).
x + y
There are six types of operator in Java programming:
- Artimatic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- and Ternary Operators
Arithmetic operators are operators that we usually find for mathematical operations. Arithmetic itself is a branch of mathematics which discusses simple calculations such as times, divide, add and less.
Apart from these four operations, the Java language also has the modulo division operation, or operator % which is used to find the remainder of the quotient.
The following table summarizes the arithmetic operators in the Java programming language:
NAME | SYMBOL |
Addition | + |
Substraction | - |
Multiplication | * |
Division | / |
Modulus | % |
How to use it?
Create a new class named Arithmetic Operators, then type following code:
import java.util.Scanner;
public class ArithmeticOperators {
public static void main(String[] args) {
int num1;
int num2;
int result;
Scanner keyboard = new Scanner(System.in);
System.out.print("Input number 1: ");
num1 = keyboard.nextInt();
System.out.print("Input number 2: ");
num2 = keyboard.nextInt();
// addition
result = num1 + num2;
System.out.println("Result = " + result);
System.out.print("Input number 1: ");
num1 = keyboard.nextInt();
System.out.print("Input number 2: ");
num2 = keyboard.nextInt();
// subtraction
result = num1 - num2;
System.out.println("Result = " + result);
System.out.print("Input number 1: ");
num1 = keyboard.nextInt();
System.out.print("Input number 2: ");
num2 = keyboard.nextInt();
// multiplication
result = num1 * num2;
System.out.println("Result = " + result);
System.out.print("Input number 1: ");
num1 = keyboard.nextInt();
System.out.print("Input number 2: ");
num2 = keyboard.nextInt();
// Division
result = num1 / num2;
System.out.println("Result = " + result);
System.out.print("Input number 1: ");
num1 = keyboard.nextInt();
System.out.print("Input number 1: ");
num2 = keyboard.nextInt();
// Remainder
result = num1 % num2;
System.out.println("Result = " + result);
}
}
Program result:
Input number 1: 3
Input number 2: 4
Result = 7
Input number 1: 6
Input number 2: 4
Result = 2
Input number 1: 3
Input number 2: 4
Result = 12
Input number 1: 8
Input number 2: 2
Result = 4
Input number 1: 24
Input number 1: 5
Result = 4
2.Assignment Operator
Assignment operators are operators that are used to assign values to a variable. In Java, the assignment operator uses an equal sign "=". Later there will also be compound assignment operators, such as "+ =", "- =", etc.
Example:
int a = 10;
The variable a is assigned to store the value 10.
The Assignment Operators consist of:
OPERATOR | EXAMPLE | SAME AS |
= | x = 5 | x = 5 |
+= | x += 3 | x = x + 3 |
-= | x -= 3 | x = x - 3 |
*= | x *= 3 | x = x * 3 |
/= | x /= 3 | x = x / 3 |
%= | x %= 3 | x = x % 3 |
&= | x &= 3 | x = x & 3 |
|= | x |= 3 | x = x | 3 |
^= | x ^= 3 | x = x ^ 3 |
>>= | x >>= 3 | x = x >> 3 |
<<= | x <<= 3 | x = x << 3 |
Now, create a new class named Assignment Operator.
public class AssignmentOperator {
public static void main(String[] args) {
int a;
int b;
// value assignment
a = 5;
b = 10;
// addition
b += a;
// now b = 15
System.out.println("Addition : " + b);
// subtraction
b -= a;
// now b = 10 (15-5)
System.out.println("Subtraction : " + b);
// multiple
b *= a;
// now b = 50 (10*5)
System.out.println("Multiple : " + b);
// Division
b /= a;
// now b=10
System.out.println("Division : " + b);
// Remainder
b %= a;
// now b=0
System.out.println("Remainder: " + b);
}
}
Program result:
Addition : 15
Subtraction : 10
Multiple : 50
Division : 10
Remainder: 0
3. Comparison Operators
The comparison operator is used to compare 2 values, whether the values are equal, smaller, larger, etc.. The result of this comparison operator is a boolean True or False.
The following table summarizes the results of the comparison operators in Java:
Operator | Explanation | Example | Result |
== | Equal to | 5 == 5 | true |
!= | Not equal | 5 != 5 | false |
> | Greater than | 5 > 6 | false |
< | Less than | 5 < 6 | true |
>= | Greater than or equal to | 5 >= 3 | true |
<= | Less than or equal to | 5 <= 5 | true |
The following is an example of a comparison operator program code in Java:
public class LearnJava {
public static void main(String args[]){
int a = 10;
int b = 5;
boolean result;
result = a == b;
System.out.println("a == b? " + result );
result = a != b;
System.out.println("a != b ? " + result );
result = a > b;
System.out.println("a > b ? " + result );
result = a < b;
System.out.println("a < b ? " + result );
result = a >= b;
System.out.println("a >= b ? " + result );
result = a <= b;
System.out.println("a <= b ? " + result );
}
}
Program result:
a == b? false
a != b ? true
a > b ? true
a < b ? false
a >= b ? true
a <= b ? false
4. Logical Operators
Logical operators are used to return true or false boolean values of 2 or more conditions. The following table summarizes the results of the logical operators in the Java language:
Operator | Name | Description | Example |
&& | Logical and | Returns true if both statements are true | x < 5 && x < 10 |
|| | Logical or | Returns true if one of the statements is true | x < 5 || x < 4 |
! | Logical not | Reverse the result, returns false if the result is true | !(x < 5 && x < 10) |
public class LearnJava {
public static void main(String args[]){
boolean a = true;
boolean b = false;
boolean result;
result = a && b;
System.out.println("Result a && b : " + hasil );
result = a || b;
System.out.println("Result a || b : " + hasil );
result = !b;
System.out.println("Result !b : " + hasil );
}
}
Program result:
Result a && b : false
Result a || b : true
Result !b : true
5. Bitwise Operators
Bitwise operators are operators used for bit (binary) operations.
Bitwise operators consist of:
Name | Symbol |
AND | & |
OR | | |
NOT | ~ |
XOR | ^ |
Zero-fill left shift | << |
Signed right shift | >> |
Zero-fill right shift | >>> |
The concept is almost the same as the Logic operator. The difference is, Bitwise is used for binary.
Let's try it in a program..Create a new class with the name OptBitwise, then copy below code:
public class OptBitwise {
public static void main(String[] args) {
int a = 60; /* 60 = 0011 1100 */
int b = 13; /* 13 = 0000 1101 */
int c = 0;
c = a & b; /* 12 = 0000 1100 */
System.out.println("a & b = " + c);
c = a | b; /* 61 = 0011 1101 */
System.out.println("a | b = " + c);
c = a ^ b; /* 49 = 0011 0001 */
System.out.println("a ^ b = " + c);
c = ~a; /*-61 = 1100 0011 */
System.out.println("~a = " + c);
c = a << 2; /* 240 = 1111 0000 */
System.out.println("a << 2 = " + c);
c = a >> 2; /* 215 = 1111 */
System.out.println("a >> 2 = " + c);
c = a >>> 2; /* 215 = 0000 1111 */
System.out.println("a >>> 2 = " + c);
}
}
Program result:
a & b = 12
a | b = 61
a ^ b = 49
~a = -61
a << 2 = 240
a >> 2 = 15
a >>> 2 = 15
6. Ternary Operators
The ternary operator is an operator that consists of 3 operands. In Java, this ternary operator uses a question mark (?)aAnd a colon (:) to separate the answer.
Here is an example of using ternary operators in the Java programming language:
class OptTernary {
public static void main(String[] args) {
boolean like = true;
String answer;
// using ternary operators
answer = like ? "yes" : "no";
// displays answers
System.out.println(answer);
}
}
Program result:
yes
We have studied the various types of operators in Java programming.
Yes, operators are very closely related to mathematics. Therefore, programmers are required to be able to do mathematics.