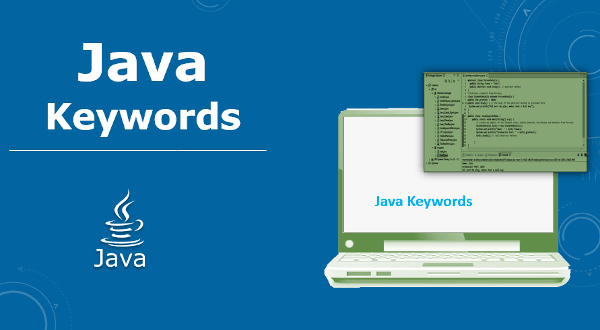
A java application is a group of classes that talk to each other by executing other class methods and sending messages by entering parameters into the method. In this section we will learn about java keywords, identifers and the concept of access control.
What is java keyword?
Java only has 44 keywords. All of these keywords belong to the Java language. So, we must use it correctly and should not be used for other purposes, for example as variable names or class names.
The following is a list of 44 java keywords:
abstract | Boolean | break | byte | case | catch |
char | class | const | continue | default | do |
double | else | extends | final | finally | float |
for | goto | if | implements | import | instanceof |
int | interface | long | native | new | package |
private | protected | public | return | short | static |
strictfp | super | switch | synchronized | this | throw |
throws | transient | try | void | volatile | while |
assert | enum |
Some keywords are quite familiar in all programming languages, so there is no need to explain in detail.
What is java identifiers?
Identifers are names that can be declared in java but are not part of the java keyword. Java identifers include: classes, interfaces, variables/properties and methods.
The procedure for naming identifers in java is governed by several rules:
- The rules of the compiler to determine whether the name identifers are allowed or not.
- Java Code Convention from Sun.
- JavaBean naming standard.
The rules of the compiler about naming identifiers are very clear because there will be an error at compile time if this rule is violated.
Following are the naming rules for identifiers used by compilers:
- All java keywords may not be used as identifiers.
- Identifiers must begin with a letter, a dollar symbol ($) or an underscore connecting character (_). Numbers cannot be used as the first character identifiers.
- After the first character, the next may be followed by letters, dollar symbols, connecting characters, and numbers.
- There are no restrictions on the length of identifiers.
- Java identifiers are case-sensitive, foo and Foo are two different identifiers.
- The public class name must exactly match the name file .java
- int _x;
- int $y;
- int ___17_r;
- int _$;
- int this_is_identifiers_name;
- int 123test_test;
- int x#;
- int x:;
- int x:;
- int .point;
Following are some of the conventions used in the Java Code Convention:
- Classes and interfaces always start with a capital letter. Every word always starts with a capital letter. This style is commonly called the "Camel Case". For example: Runnable, HashMap, ArrayList, and so on. Also, classes must be nouns, not adjectives or verbs.
- Methods always start with a lowercase letter. Every word after the first letter starts with an uppercase letter. The method must be a verb to indicate that this is doing an activity/action. For example: getIndex, setIndex, println, paint, and so on.
- Just like methods, variables using camel cases start with lowercase letters. Variables should be short, clear, sound good, and nouns. For example: index, length, width, firstIndex, and so on.
- Constants in java are created by declaring a variable as static and final. All letters are uppercase letters separated by underscore (_) symbols. For example: FRAME_WIDTH, ERROR_MESSAGE, and so on.
Modern frameworks such as Spring and EJB have also adopted the concept of JavaBean, so the term JavaBean often appears in the documentation of this framework. Spring uses the term bean instead of JavaBean, but technically they are the same.
To understand the concept of JavaBean, there is a term called Properties. Basically, a property is an instance variable that is directly below the class, whose access modifer is private. Since it is private, a method must be made to access properties from outside the class. The method for accessing properties is usually referred to as a getter and the method for changing property values is called a setter.
The following rules for naming methods are used to access the properties (getter setter) of JavaBean:
- If the data property type is not boolean, then the method for accessing properties starts with get. for example getWidth, getSize, getIndex and so on.
- If the data property type is boolean, then the method for accessing properties starts with is. For example isEmpty, isRunning and so on.
- All setter methods must start with a set. For example setSize, setIndex, setWidth and so on
- The method name is derived from the variable name that is given the prefix get, set, or is. Camel case writing rules apply to the getter and setter methods.
- Setter methods must be public, return void with one parameter whose data type is exactly the same as the variable data type.
- Setter methods must be public, return data types that are the same as variable data types, and without parameters.
- JavaBean must have a default constructor, that is, a constructor that has no parameters at all.
Java Access Modifier
Access Modifier is an "access right" given to a variable, method or class that aims to maintain the integrity of data when it wants to be accessed by other objects. The access right is given by the program maker. With the Access Modifier, we can limit which resources can be accessed by certain objects, their derivatives, or by certain methods.
In the Java programming language, there are four types of modifiers can be used for variables, methods and classes. The following is a table that briefly explains the types and levels of permissions on the modifier:
Modifier | Class | Package | Subclass | World |
No Modifier | Y (Accessible) | Y (Accessible) | N (Not accessible) | N (Not accessible) |
public | Y (Accessible) | Y (Accessible) | Y (Accessible) | Y (Accessible) |
protected | Y (Accessible) | Y (Accessible) | Y (Accessible) | N (Not accessible) |
private | Y (Accessible) | N (Not accessible) | N (Not accessible) | N (Not accessible) |
Public access modifier means that it can be accessed by anyone without restrictions.
Notice the example below:
The fruit class is declared public and within the com.mypackage package.
package com.mypackage;
public class Fruit {
private String name;
public void setFruitName(String fruitName) {
this.name = fruitName;
}
public String getFruitName() {
return name;
}
public void showMessage(){
System.out.println("The name of the fruit is: " + getFruitName());
}
}
Then the FruitTest class is in the test package as below.
package test;
//import statement to use classes outside the package
import com.mypackage.Fruit;
public class FruitTest {
public static void main(String args []){
Fruit fruit = new Fruit();
fruit.setFruitName("Grape");
fruit.showMessage();
}
}
Note that the Fruit.class must be declared public so it can be used by the FruitTest.class where it is in a different package. If this is not the case, then the Fruit.class is considered to have a default access modifier where it can only be accessed in the same package. So an error occurs stating that the Fruit.class is "not visible".
Protected means that it can be accessed inside the package or outside the package but only through inheritance which is one of the important concepts of java programming.
Protected cannot be applied to classes, but can be applied to data fields, methods and constructors.
Consider the following simple example:
The Fruit.class resides in the com.mypackage and applies the protected modifier to the showMessage(); method.
package com.mypackage;
public class Fruit {
protected void showMessage(String color){
System.out.println("The color of the fruit is: " + color);
}
}
Apple.class is in the test package and inherits the Fruit.class (using the keyword extends). Here the object of the Apple.class uses the method showMessage() from the Fruit.class.
package test;
import com.mypackage.Fruit;
public class Apple extends Fruit {
private String color = "Red";
public static void main(String args []){
Apple apple = new Apple();
apple.showMessage(apple.color);
}
}
The last is a Private access modifier. This is the most restrictive modifier acces. Private means that methods and variables can only be accessed by the same class. An instance variable with a private access modifier can only be used by methods in the same class, but cannot be seen or used by other classes or objects.
Private methods can be called by other methods in the same class but cannot be called by other classes. In addition, either the method or the private variable cannot be inherited or inherited from the subclass.
Consider the example below, where the Fruit.class and the FruitTest.class are in the same package which is com.mypackage. The showMessage() method has a default access modifier. When this method is to be accessed by the FruitTest.class (different class), an error will occur stating that the method is "not visible".
package com.mypackage;
public class Fruit {
String name = "Grape";
private void showMessage(){
System.out.println("The name of the fruit is: " + name );
}
}
package com.mypackage;
public class FruitTest {
public static void main(String args []){
Fruit fruit = new Fruit();
//instance variable name from the Fruit class has default modifier
//so that it can be accessed in the same package by different classes
System.out.print(fruit.name);
//The method showMessage not visible because private modifier
fruit.showMessage();//Error occured
}
}
Private can be used in class constructors. However, if it is implemented, you can not create an object outside the class.
That's all about the difference between public, private, and protected modifiers in Java. Rule of thumb is to keep things as much private as possible because that allows you the flexibility to change them later. If you cannot make them private then at-least keep them package-private, which is also the default access level for class, methods, and variables in Java. You should also keep in mind that access modifiers can only be applied to members e.g. variables and methods which are part of the class, you can not make a local variable public, private, or protected in Java.