
Branching is also known as "Control Flow", "Condition Structure", "IF Structure", "Decision", etc. Everything is the same.
Generally, the flow of code program execution is done one by one from top to bottom.
Line by line read, then the computer does as instructed.
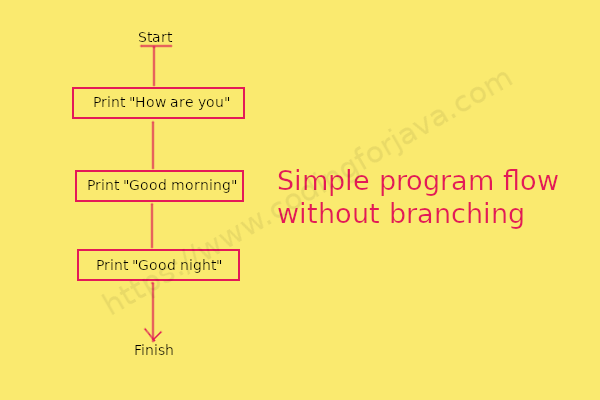
In the diagram above, the flow is indeed one.
But after we use the branching, the flow will increase like this.

Then how to write branching statements in Java?
Branching statements in java language is divided into:
- if (simple)
- if-else
- if-else-if
- switch case
If Statement
This branching has only one choice. Means, IF option will only be executed if true. Examples of IF structure formats like this:
if (kondisi){
statement1;
statement2;
}
Statement1 and statement2 will be executed when the condition is true. Meanwhile, when the condition is false, both statements are not executed by the program.
As an example, let's create a simple java program.
Suppose there is a bookstore. They give gifts of school supplies to buyers who are spending over USD 100.
Then we can make a program like this:
import java.util.Scanner;
public class DoorPrize {
public static void main(String[] args) {
// make shopping variables and scanner
int shopping = 0;
Scanner scan = new Scanner(System.in);
// take user input
System.out.print("Total Expenditures: $ ");
shopping = scan.nextInt();
// check whether the buyer shopping above 100
if ( shopping > 100 ) {
System.out.println("Congratulations, you get a prize!!");
}
System.out.println("Thank you...");
}
}
Run the program and watch the results.

Try to give a value under 100 and watch what happens.
IF ELSE statement
In the second IF branch there is an ELSE section where there are conditions that are not met, then a statement on the ELSE section will be executed. Examples of IF structure formats like this:
if (condition){
statement1;
statement2;
}else {
alternative_statement1;
alternative_statement2;
}
Here, alternative_statement1 and alternative_statement2 inside the ELSE section will be run when the condition is false.
As an example, let's create a simple student grade java program.
For example, if a student's grade is greater than 70, then he is declared graduated. If not, then he fails.
We can make the program like this:
import java.util.Scanner;
public class StudentGrade {
public static void main(String[] args) {
// create a variable and Scanner
int grade;
String name;
Scanner scan = new Scanner(System.in);
// take user input
System.out.print("Name: ");
name = scan.nextLine();
System.out.print("Grade: ");
grade = scan.nextInt();
// check whether students graduate or not
if( grade >= 70 ) {
System.out.println("Congratulations " + name + ", you have successfully graduated!");
} else {
System.out.println("Sorry " + name + ", you failed");
}
}
}
Run the program and watch the results.

Try to change the input values and see what will happen.
IF ELSE IF statement
if-else-if statement is used when we need to check multiple conditions. In this statement we have only one “if” and one “else”, however we can have multiple “else if”. It is also known as if else if ladder. This is how it looks:
if (condition1) {
statement1;
statement2;
} else if (condition2) {
statement3;
statement4;
} else if (condition3) {
statement5;
statement6;
} else {
alternative_statement;
}
We can see above, it has 3 conditions. The program will read the condition from top to bottom sequentially, where condition1 will be checked whether it is true? If Yes then statement1 and statement2 will be executed.
If the condition is false, it will be checked for condition2 and so on, until the condition3. If all conditions are false, then the program will execute an alternative statement in the ELSE section.
Note: The most important point to note here is that in if-else-if statement, as soon as the condition is met, the corresponding set of statements get executed, rest gets ignored. If none of the condition is met then the statements inside “else” gets executed.
Example java program using if else if statement:
import java.util.Scanner;
public class StudentGrade {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
int grade;
System.out.print("Enter Value : ");
grade = sc.nextInt();
if (grade>=90){
System.out.println("Very good");
}else if (grade>=80){
System.out.println("Good");
}else if (grade>=70){
System.out.println("Average");
}else if (grade>=60){
System.out.println("Minus");
}else {
System.out.println("Worse");
}
}
}
The above program makes a branching with several statements to be executed only if the conditions are met. There, I create a grade variable for the user input.
The condition checked first is the condition at the top. If the value entered is more than 90? then the output is "very good". If the condition is not met, the program will continue to the next statement where the condition is true.
Below is the program output with various grade input.

SWITCH CASE statement
A switch statement allows a variable to be tested for equality against a list of values. Each value is called a case, and the variable being switched on is checked for each case. The syntax structure is below:
switch (expression){
case value:
statement1;
break;
case value:
statement2;
break;
case value:
statement3;
break;
default :
default_statement;
}
Example java program using SWITCH CASE statement:
import java.util.Scanner;
public class Faculty {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
int facultyCode;
System.out.print("Enter Faculty Code : ");
facultyCode = sc.nextInt();
switch(facultyCode) {
case 1:
System.out.println("Information Management");
break;
case 2:
System.out.println("Computer Engineering");
break;
case 3:
System.out.println("Accounting");
break;
case 4:
System.out.println("International Law");
break;
case 5:
System.out.println("Philosophy");
break;
default:
System.out.println("Sorry, unavailable!!!!!!");
}
}
}
The program output with various input entered:

We have already learned several types of branching in java.
The summary is below:
- IF, only have one choice;
- IF / ELSE has two choices;
- IF / ELSE / IF has more than two choices;
- SWITCH / CASE is another form of IF / ELSE / IF;
- Nested branching is a branching in a branching;
- The use of logical operators in branching can make branching shorter.